Remix Installation
Install and configure OnchainKit with Remix. If you are integrating OnchainKit into an existing project, skip to the OnchainKit installation.
Install Remix
Create a new Remix project by using the Remix CLI. More information about Remix can be found here.
npx create-remix@latest
Install OnchainKit
Add OnchainKit to your project by installing the @coinbase/onchainkit
package.
npm install @coinbase/onchainkit
Get A Client API Key
Get your Client API Key from Coinbase Developer Platform.
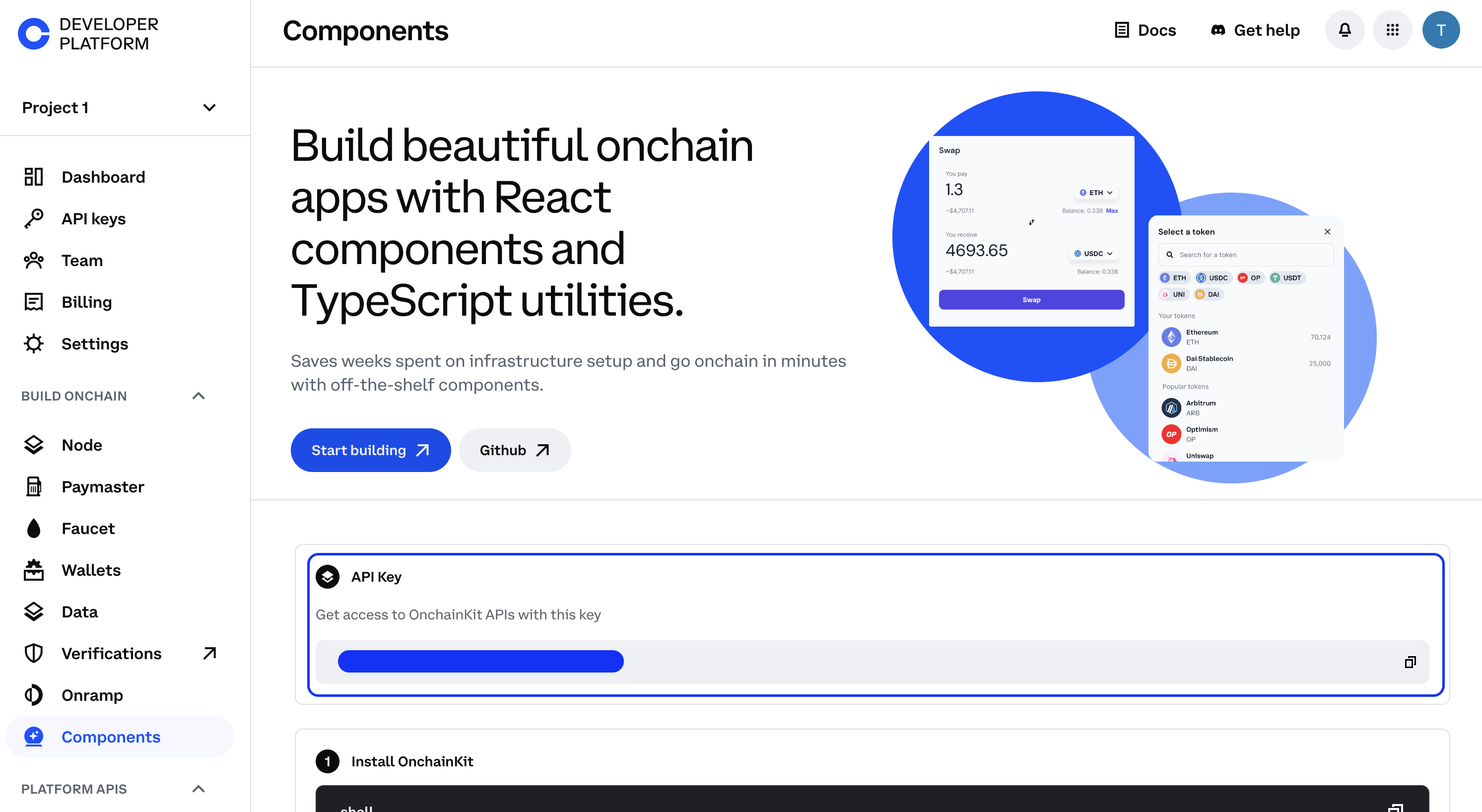
Create a .env
file in your project's root directory.

Add your Client API Key to the .env
file:
PUBLIC_ONCHAINKIT_API_KEY=YOUR_CLIENT_API_KEY
Update the app/root.tsx
file to provide access to your Client API Key
through window.ENV
:
declare global {
interface Window {
ENV: {
PUBLIC_ONCHAINKIT_API_KEY: string;
};
}
}
export async function loader() {
return json({
ENV: {
PUBLIC_ONCHAINKIT_API_KEY: process.env.PUBLIC_ONCHAINKIT_API_KEY,
},
});
}
If this is the first env variable you've added to your project, you will need to
update the Layout
component of app/root.tsx
to make it available to your app:
export function Layout({ children }: { children: React.ReactNode }) {
const data = useLoaderData<typeof loader>();
return (
<html lang="en">
<head>
<meta charSet="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<Meta />
<Links />
</head>
<body>
{children}
<ScrollRestoration />
<script
dangerouslySetInnerHTML={{
__html: `window.ENV = ${JSON.stringify(
data.ENV
)}`,
}}
/>{' '}
<Scripts />
</body>
</html>
);
}
Add Providers
Create a providers.tsx
file. Add OnchainKitProvider
with your desired config.
Under the hood, OnchainKit will create our recommended Wagmi and QueryClient providers. If you wish to customize these providers, check out Custom Supplemental Providers.
'use client';
import type { ReactNode } from 'react';
import { OnchainKitProvider } from '@coinbase/onchainkit';
import { base } from 'wagmi/chains'; // add baseSepolia for testing
export function Providers(props: { children: ReactNode }) {
const apiKey =
typeof window !== 'undefined'
? window.ENV?.PUBLIC_ONCHAINKIT_API_KEY
: undefined;
return (
<OnchainKitProvider
apiKey={apiKey}
chain={base} // add baseSepolia for testing
>
{props.children}
</OnchainKitProvider>
);
}
Wrap your app with <Providers />
After configuring the providers in step 4, wrap your app with
the <Providers />
component.
import { Providers } from 'src/Providers';
export default function App() {
return (
<Providers>
<Outlet />
</Providers>
);
}
Import OnchainKit Styles
OnchainKit components come with pre-configured styles.
To include these styles in your project, add the following import
statement at the top of the file where imported <Providers />
:
import { Providers } from 'src/Providers';
import '@coinbase/onchainkit/styles.css';
export default function App() {
return (
<Providers>
<Outlet />
</Providers>
);
}
This ensures that the OnchainKit styles are loaded and applied to your entire application.
-
For Tailwind CSS users, check out our Tailwind Integration Guide.
-
Update the appearance of components by using our built-in themes or crafting your own custom theme. Explore the possibilities in our Theming Guide.
Start building!
Identity
- Show Basenames, avatars, badges, and addresses.Wallet
- Create or connect wallets with Connect Wallet.Transaction
- Handle transactions using EOAs or Smart Wallets.Checkout
- Integrate USDC checkout flows with ease.Fund
- Create a funding flow to onboard users.Tokens
- Search and display tokens with various components.Swap
- Enable token swaps in your app.Mint
- View and Mint NFTs in your app.